Ampelschaltung als Moore-Automat
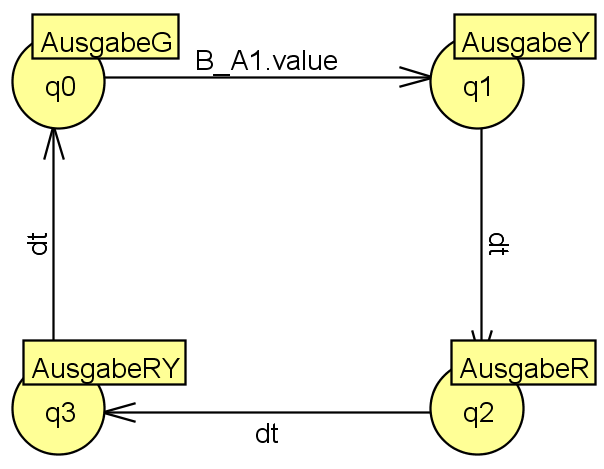
import board
import time
import neopixel
import touchio
NeopixelReihe = neopixel.NeoPixel(board.NEOPIXEL, 10)
NeopixelReihe.auto_write = False
B_A1 = touchio.TouchIn(board.A1)
zustand = "q0"
startzeit = time.monotonic()
dt = time.monotonic()
AusgabeR = [(16, 0, 0), (0, 0, 0), (0, 0, 0)]
AusgabeY = [(0, 0, 0), (16, 16, 0), (0, 0, 0)]
AusgabeG = [(0, 0, 0), (0, 0, 0), (0, 16, 0)]
AusgabeRY = [(16, 0, 0), (16, 16, 0), (0, 0, 0)]
def resetTimer():
global startzeit
startzeit = time.monotonic()
def macheAusgabe(zustand):
if zustand == "q0":
NeopixelReihe[2] = AusgabeG[0]
NeopixelReihe[1] = AusgabeG[1]
NeopixelReihe[0] = AusgabeG[2]
if zustand == "q1":
NeopixelReihe[2] = AusgabeY[0]
NeopixelReihe[1] = AusgabeY[1]
NeopixelReihe[0] = AusgabeY[2]
if zustand == "q2":
NeopixelReihe[2] = AusgabeR[0]
NeopixelReihe[1] = AusgabeR[1]
NeopixelReihe[0] = AusgabeR[2]
if zustand == "q3":
NeopixelReihe[2] = AusgabeRY[0]
NeopixelReihe[1] = AusgabeRY[1]
NeopixelReihe[0] = AusgabeRY[2]
NeopixelReihe.show()
def zustandsuebergangsfunktion():
global zustand
global dt
global startzeit
if zustand == "q0":
macheAusgabe(zustand)
if B_A1.value:
zustand = "q1"
resetTimer()
return
elif zustand == "q1":
macheAusgabe(zustand)
if dt > 1.0:
resetTimer()
zustand = "q2"
return
elif zustand == "q2":
macheAusgabe(zustand)
if dt > 1.0:
resetTimer()
zustand = "q3"
return
elif zustand == "q3":
macheAusgabe(zustand)
if dt > 1.0:
resetTimer()
zustand = "q0"
return
while True:
zustandsuebergangsfunktion()
dt = time.monotonic() - startzeit
time.sleep(0.1)